AI Brain is a computational framework designed to mimic human cognitive functions such as perception, reasoning, memory, learning, and decision-making. Unlike traditional AI systems that follow predefined rules, an AI Brain adapts, learns, and autonomously makes decisions by integrating multiple AI models and algorithms.
An AI Brain is the next evolution of AI systems, integrating perception, memory, reasoning, learning, and action into a unified framework. Using LLMs, LangGraph, memory retrieval, and reinforcement learning, we can build adaptive AI assistants and autonomous agents.
- Hyper-Personalized AI Agents (AI that remembers everything about you)
- Self-Learning AI (AI that improves over time without retraining)
- Neuro-Symbolic AI (Combining symbolic logic with deep learning)
- AGI (Artificial General Intelligence) (Fully autonomous AI with human-like reasoning)
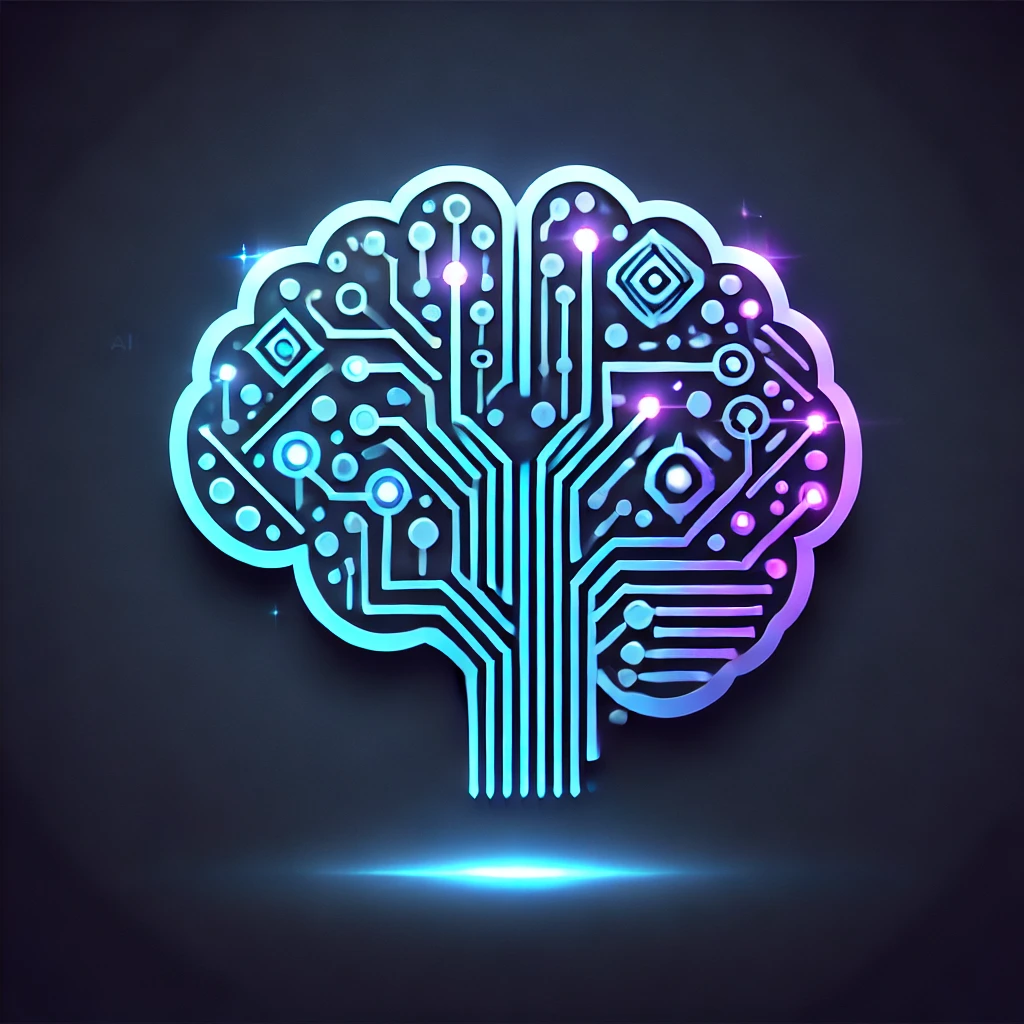
Key Components of an AI Brain
To build an AI Brain, we need the following core components:
1. Perception Layer (Input Processing)
- Function: Gathers and processes input data from multiple sources (text, images, voice, sensors).
- Models Used:
- NLP Models (GPT-4, LLaMA, Claude) – Text processing
- Computer Vision (YOLO, CLIP, ViT) – Image recognition
- Speech Recognition (Whisper, Wav2Vec) – Audio processing
2. Memory Layer (Short-term & Long-term Memory)
- Function: Stores past interactions and retrieves relevant context.
- Types of Memory:
- Short-term Memory: Keeps recent conversation context.
- Long-term Memory: Uses Vector Databases (FAISS, Pinecone) to store embeddings of past knowledge.
- Models Used:
- LangChain + Vector Databases (Pinecone, Weaviate) for retrieval-augmented generation (RAG).
- Transformer Embeddings (OpenAI, BERT, Sentence Transformers) for contextual search.
3. Reasoning Layer (Logical Thinking & Analysis)
- Function: Uses LLMs to analyze, infer, and generate responses.
- Models Used:
- LLMs (GPT-4, Mistral, Claude, Gemini) – Text-based reasoning
- Graph-based AI (LangGraph, DeepMind AlphaZero) – Multi-step logical reasoning
4. Learning Layer (Self-Improvement)
- Function: Adapts and updates knowledge dynamically.
- Approaches:
- Reinforcement Learning (RLHF, PPO, AlphaGo)
- Fine-tuning LLMs on new data
- Self-learning models with AutoML
- Models Used:
- Deep Q-Networks (DQN), PPO (OpenAI Gym, Stable-Baselines3)
- Self-improving LLMs (LoRA, PEFT fine-tuning)
5. Decision-Making Layer (Planning & Execution)
- Function: Selects the best course of action based on goals and input.
- Techniques Used:
- Symbolic AI (Knowledge Graphs, Neo4j, ConceptNet)
- Multi-Agent AI (LangGraph, AutoGPT, BabyAGI)
- Decision Trees & Bayesian Networks
- Models Used:
- OpenAI Functions + ReAct Framework (for planning)
- Multi-Agent LLM Collaboration (ChatDev, CrewAI)
6. Action Layer (Task Execution)
- Function: Executes actions based on AI Brain decisions, such as:
- Answering questions
- Automating workflows
- Controlling IoT devices
- Models Used:
- LLM Agents (LangChain, AutoGPT, CrewAI)
- API Call Automation (Zapier, LangChain Tools)
How to Build an AI Brain
Step 1: Define the AI Brain Architecture
We structure the AI Brain as a modular system where each component interacts.
Step 2: Implement Perception (LLMs, Vision, Speech)
Example: Processing user input with an LLM
pythonCopyEditfrom langchain.chat_models import ChatOpenAI
llm = ChatOpenAI(model="gpt-4")
response = llm.predict("What is the future of AI?")
print(response)
Step 3: Add Memory (Vector Databases)
Example: Storing knowledge for long-term recall
pythonCopyEditfrom langchain.vectorstores import FAISS
from langchain.embeddings import OpenAIEmbeddings
vectorstore = FAISS.from_texts(["AI memory storage is crucial."], OpenAIEmbeddings())
retrieved_docs = vectorstore.similarity_search("Tell me about AI memory")
print(retrieved_docs)
Step 4: Implement Reasoning & Decision-Making
Example: Using LangGraph for reasoning
pythonCopyEditfrom langgraph.graph import StateGraph
from typing import TypedDict, List
class BrainState(TypedDict):
messages: List[str]
memory: List[str]
def reasoning(state: BrainState) -> BrainState:
latest_input = state["messages"][-1]
response = llm.predict(f"Analyze this: {latest_input}")
return {"messages": state["messages"] + [response], "memory": state["memory"]}
brain = StateGraph(BrainState)
brain.add_node("reasoning", reasoning)
brain.set_entry_point("reasoning")
ai_brain = brain.compile()
output = ai_brain.invoke({"messages": ["What is AI?"], "memory": []})
print(output["messages"])
Step 5: Enable Learning (Self-Improvement)
Example: Fine-tuning LLM responses dynamically
pythonCopyEditfrom transformers import AutoModelForCausalLM, AutoTokenizer
model = AutoModelForCausalLM.from_pretrained("mistral-7b")
tokenizer = AutoTokenizer.from_pretrained("mistral-7b")
input_text = "Teach me about AI reasoning."
inputs = tokenizer(input_text, return_tensors="pt")
output = model.generate(**inputs)
print(tokenizer.decode(output[0]))